How to Interface an I2C 16×2 LCD with Arduino Uno
Are you looking to simplify your Arduino projects while adding a sleek LCD display? An I2C 16×2 LCD module is a game-changer. With just two data pins required for communication, it’s a compact and cost-effective way to add a visual element to your project. This guide walks you through the process of connecting and coding an I2C LCD display with an Arduino Uno to display scrolling messages.
What is an I2C 16×2 LCD Display?
An I2C 16×2 LCD is a liquid crystal display with an I2C backpack module that enables simple communication using the I2C protocol. It can display 16 characters per row across 2 rows, perfect for small projects that need text-based outputs.
Why Choose an I2C 16×2 LCD?
Advantages
– Simplified Wiring: Requires only two data pins (SDA and SCL), reducing clutter.
– Compact Design: Ideal for projects with space constraints.
– Budget-Friendly: Low-cost solution for text displays.
– Easy Coding: Supported by well-documented libraries like LiquidCrystal_I2C.
Disadvantages
– Slower Communication: I2C protocol may not suit high-speed applications.
– Limited Display Size: Restricted to 16×2 characters.
– Basic Graphics: No support for custom colours or advanced graphics.
What You’ll Need
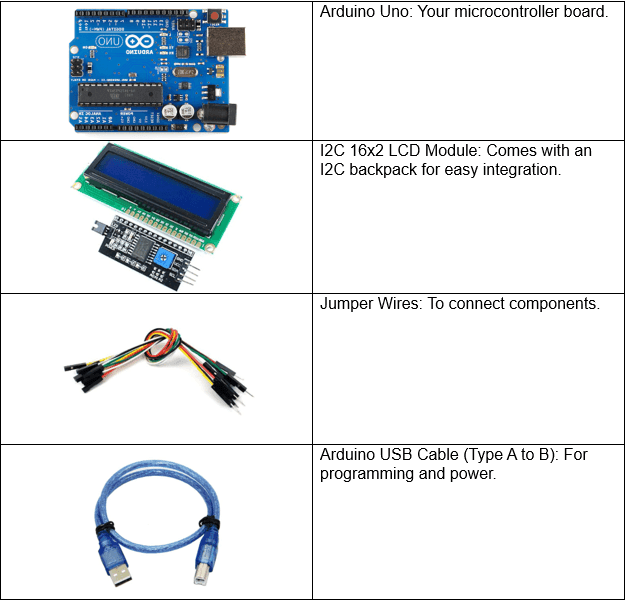
Pin Configuration of I2C 16×2
The I2C LCD module has four pins:
– VCC: Connects to the 5V power supply.
– GND: Connects to ground.
– SDA (Serial Data): Transfers data.
– SCL (Serial Clock): Sends clock signals.
Connection Guide
I2C LCD Pin | Arduino Uno Pin |
VCC | 5V |
GND | GND |
SDA | A4 |
SCL | A5 |
Step-by-Step Wiring Instructions
1. Connect Power
– Attach the VCC pin of the LCD to the 5V pin on the Arduino.
– Connect the GND pin of the LCD to the GND pin on the Arduino.
2. Connect Data Pins
– Connect the SDA pin of the LCD to A4 on the Arduino.
– Connect the SCL pin of the LCD to A5 on the Arduino.
Coding for Scrolling Messages
Use the LiquidCrystal_I2C library for simple control of the LCD. Below is a sample code to scroll custom messages.
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
// Set the LCD address (default is 0x27; verify your module)
LiquidCrystal_I2C lcd(0x27, 16, 2);
void setup() {
lcd.begin(); // Initialize the LCD
lcd.backlight(); // Turn on the backlight
}
void loop() {
// Display and scroll two different messages
scrollMessage("HELLO, INNOVATOR!", 300);
scrollMessage("FOLLOW @ROBOSCHOOL!", 300);
}
// Function to scroll messages
void scrollMessage(String message, int delayTime) {
lcd.setCursor(16, 0); // Start at the end of the first row
lcd.print(" "); // Print spaces for the scroll effect
for (int position = 0; position < message.length() + 16; position++) {
lcd.setCursor(0, 0);
lcd.print(message.substring(position < message.length() ? position : message.length() - 1, position + 16));
delay(delayTime);
}
}
Key Benefits of This Project
– Learn I2C Communication: Gain insights into interfacing devices with minimal pins.
– Practical Display: Use scrolling text for real-time feedback in your projects.
– Beginner-Friendly: Requires only basic components and coding knowledge.
Frequently Asked Question
How do I find the I2C address of my LCD?
Use the I2C Scanner sketch available online to determine your module’s address (commonly 0x27 or 0x3F).
Can I use other Arduino boards with this setup?
Yes, the setup is compatible with most Arduino boards. Ensure you connect SDA and SCL to the correct pins for your specific board.
Can I customise the scrolling speed?
Absolutely! Adjust the delay time parameter in the scroll Message function to change the speed.
Conclusion
Integrating an I2C 16×2 LCD with an Arduino Uno is a simple yet powerful way to enhance your projects. From displaying basic information to scrolling dynamic messages, this project is an excellent starting point for hobbyists and students alike.
Add this project to your DIY electronics arsenal, and start impressing your friends with professional-looking outputs!
Ready to build? Share your results with us on social media and tag @Roboschool for a chance to be featured!
Responses